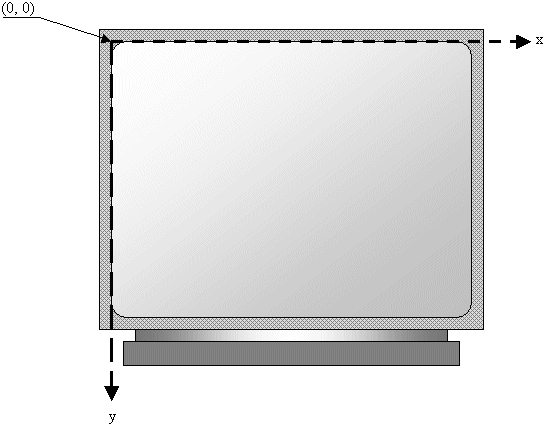
Getting a Device Context
In order to draw using a device context, you must first declare an HDC variable. This can be done as follows:
HDC hDC;
After declaring this variable, you must prepare the application to paint by initializing it with a call to the BeginPaint() function. The syntax of the BeginPaint() function is:
HDC BeginPaint(HWND hWnd, LPPAINTSTRUCT lpPaint);
The hwnd argument is a handle to the window on which you will be painting
The lpPaint argument is a pointer to the PAINTSTRUCT structure. This means that, the BeginPaint() function returns two values. It returns a device context as HDC and it returns information about the painting job that was performed. That painting job is stored in a PAINTSTRUCT value. The PAINTSTRUCT structure is defined as follows:
typedef struct tagPAINTSTRUCT {
HDC hdc;
BOOL fErase;
RECT rcPaint;
BOOL fRestore;
BOOL fIncUpdate;
BYTE rgbReserved[32];
} PAINTSTRUCT, *PPAINTSTRUCT;
After initializing the device context, you can call a drawing function or perform a series of calls to draw. After painting, you must let the operating system know by calling the EndPaint() function. Its syntax is:
BOOL EndPaint(HWND hWnd, CONST PAINTSTRUCT *lpPaint);
Painting with the BeginPaint() and EndPaint() functions must be performed in the WM_PAINT message.
Starting a Device Context's Shape
To keep track of the various drawings, the device context uses a coordinate system that has its origin (0, 0) on the top-left corner of the desktop:
Anything that is positioned on the screen is based on this origin. This coordinate system can get the location of an object using a horizontal and a vertical measurements. The horizontal measures are based on an x axis that moves from the origin to the right right direction. The vertical measures use a y axis that moves from the origin to the bottom direction:
This means that, if you start drawing something such as a line, it would start on the origin and continue where you want it to stop.